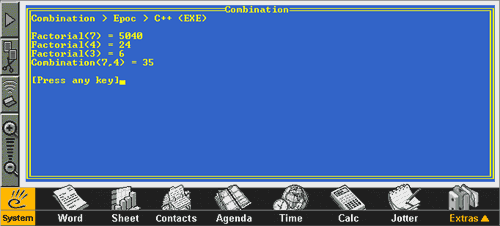
Purpose
This is the C++ version of our combination
application.
Requirements
You need Epoc C++ SDK (see tools section).
Source
code
Below is the .cpp
file.
For .dkg, .rkg,
.mmp, .bat, .exe
and README.TXT, please
download the ZIP file.
// Combination.cpp
// Epoc
// C++ (EXE)
// Includes
#include <e32base.h>
#include <e32cons.h>
// Literal definitions
_LIT(KTxtSAMPLES,"SAMPLES");
_LIT(KTxtExampleCode,"Combination");
_LIT(KFormatFailed,"Failed: leave code=%d\n");
_LIT(KTxtOK,"");
_LIT(KTxtPressAnyKey,"[Press any key]");
// Variables
LOCAL_D CConsoleBase* console; // a new console object for our output
LOCAL_C int Factorial(int n)
{
return (n <= 1) ? 1 : n*Factorial(n-1); // heh-heh... let's mix a ternary operator (?) and recursion...
}
LOCAL_C int Combination(int n, int p)
{
return Factorial(n) / (Factorial(n - p) * Factorial(p));
}
LOCAL_C void Go()
{
_LIT(KTxtTitle,"Combination > Epoc > C++ (EXE)\n");
_LIT(KTxtNewLine,"\n");
_LIT(KFormatFactorial2,"Factorial(%d) = %d\n");
_LIT(KFormatCombination3,"Combination(%d,%d) = %d\n");
console->Printf(KTxtTitle);
console->Printf(KTxtNewLine);
console->Printf(KFormatFactorial2, 7, Factorial(7));
console->Printf(KFormatFactorial2, 4, Factorial(4));
console->Printf(KFormatFactorial2, 3, Factorial(3));
console->Printf(KFormatCombination3, 7, 4, Combination(7, 4));
console->Printf(KTxtNewLine);
}
LOCAL_C void runApplication() // initialize and do main task with cleanup stack
{
console=Console::NewL(KTxtExampleCode,TSize(KConsFullScreen,KConsFullScreen));
CleanupStack::PushL(console); // push console
TRAPD(error,Go());
if (error)
console->Printf(KFormatFailed, error);
else console->Printf(KTxtOK);
console->Printf(KTxtPressAnyKey);
console->Getch(); // get and ignore character
CleanupStack::PopAndDestroy(); // close console
}
GLDEF_C TInt E32Main() // main function called by E32, equivalent of C main()
{
__UHEAP_MARK;
CTrapCleanup* cleanup=CTrapCleanup::New(); // get clean-up stack
TRAPD(error,runApplication()); // init stack and do main task
__ASSERT_ALWAYS(!error,User::Panic(KTxtSAMPLES,error));
delete cleanup; // destroy clean-up stack
__UHEAP_MARKEND;
return 0;
}
Comments
Notice that the overhead is rather tiny, compared
with version for other platforms... Also, notice that Epoc provides
the developer with a whole bunch of predefined symbols and macros
(_LIT to define a literal, LOCAL_D for local data, LOCAL_C for local
code...)
README.TXT provides useful information if you'd like
to build the app, either for WINS (the PC-based emulator) or MARM
(target processor on mobile device).
Again, the core functions are located at the beginning
of the .cpp file:
Factorial(), Combination()
and Go(). The rest of the code prepares
a clean stack, a console, invokes our Go() function, and cleans
the stuff up.
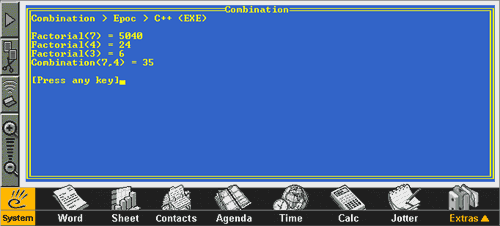
Next sample 
|