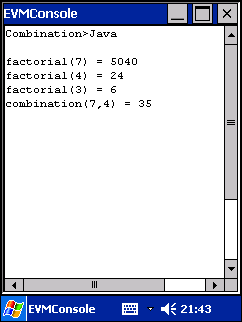
Purpose
This is the Java version of our combination
application.
Requirements
You need Java 2 SDK to compile and test the app on a desktop and Jeode
to run it on your device (see tools section).
Source
code
Below is the .java
file.
For the .class and
.bat files, please download the ZIP
file.
Please note that the .bat
file allows you to run the class on your desktop. To run the class
on your device, use your desktop's file explorer and locate \Mobile
Device\My Pocket PC\iPAQ File Store, make a Combination
folder and copy combination.jar
and combination (which
is a .bat for Pocket
PC, that defines the classpath and fires the class with 'n' and
'p' as arguments for the computation of factorial and combination).
On your device, locate \iPAQ
File Store\Combination and tap combination
(the one whose size is about 130 bytes). Feel free to place the
sample in any other folder, in which case you'll need to manually
edit the combination
file (before you copy it) and have the classpath (-cp arg) point
to whichever folder you picked.
/*
* Combination.java
* Java
*/
package samples;
/**
* A sample that computes factorial(n) and combination(n,p)
*/
public class Combination
{
private int myN, myP;
public Combination(int n, int p)
{
myN = n;
myP = p;
}
public long factorial(int n)
{
return (n <= 1) ? 1 : n*factorial(n-1); // he-he... let's mix a ternary operator (?) and recursion...
}
public long combination(int n, int p)
{
return factorial(n) / (factorial(n - p) * factorial(p));
}
public void go()
{
System.out.println("Combination>Java");
System.out.println();
System.out.println("factorial(" + myN + ") = " + factorial(myN));
System.out.println("factorial(" + myP + ") = " + factorial(myP));
System.out.println("factorial(" + (myN-myP) + ") = " + factorial(myN-myP));
System.out.println("combination(" + myN + "," + myP + ") = " + combination(myN, myP));
}
public static void main(String[] args)
{
if (args.length != 2)
{
System.err.println("Usage: Combination n p");
System.exit(0);
}
int argN = java.lang.Integer.parseInt(args[0]),
argP = java.lang.Integer.parseInt(args[1]);
Combination comb = new Combination(argN, argP);
comb.go();
}
}
Comments
This is basic Java stuff! But if portability is an
issue for your apps, it's definitely a good choice. Code above should
run as is on any platform that has a compliant Java virtual machine!
The core functions are located in factorial(),
combination() and go().
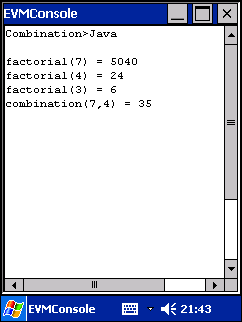
Next sample 
|