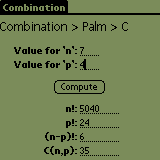
Purpose
This is the C version of our combination
application. It's one of the Palm
Samples.
Requirements
You need Palm SDK and PilRC (see tools
section).
Source
code
Below is the .c
file.
For .h, .rcp,
.bmp, Makefile and
.prc, please download
the ZIP file.
// Combination.c
// Palm
// C
#include "combination.h"
#include <palmtypes.h>
#include <PalmCompatibility.h>
#include <System/SystemPublic.h>
#include <UI/UIPublic.h>
static UInt32 Factorial(UInt32 n)
{
return (n <= 1) ? 1 : n*Factorial(n-1); // heh-heh... let's mix a ternary operator (?) and recursion...
}
static UInt32 Combination(UInt32 n, UInt32 p)
{
return Factorial(n) / (Factorial(n - p) * Factorial(p));
}
static void Go()
{
static Char* GetField(UInt16, UInt16);
static FieldPtr SetField(UInt16, UInt16, MemPtr);
UInt32 n = 0, p = 0;
Char *ptr, buf[21];
if (ptr=GetField(MainForm, MainN))
n = StrAToI(ptr);
if (ptr=GetField(MainForm, MainP))
p = StrAToI(ptr);
StrIToA(buf, Factorial(n));
SetField(MainForm, MainFactorialN, buf);
StrIToA(buf, Factorial(p));
SetField(MainForm, MainFactorialP, buf);
StrIToA(buf, Factorial(n-p));
SetField(MainForm, MainFactorialNP, buf);
StrIToA(buf, Combination(n,p));
SetField(MainForm, MainCombination, buf);
FrmDrawForm(FrmGetFormPtr(MainForm));
}
static Char* GetField(UInt16 formID, UInt16 fieldID)
{
FormPtr frm;
FieldPtr fld;
UInt16 obj;
frm = FrmGetFormPtr(formID);
obj = FrmGetObjectIndex(frm, fieldID);
fld = (FieldPtr)FrmGetObjectPtr(frm, obj);
return FldGetTextPtr(fld);
}
static FieldPtr SetField(UInt16 formID, UInt16 fieldID, MemPtr str)
{
FormPtr frm;
FieldPtr fld;
UInt16 obj;
CharPtr p;
VoidHand h;
frm = FrmGetFormPtr(formID);
obj = FrmGetObjectIndex(frm, fieldID);
fld = (FieldPtr)FrmGetObjectPtr(frm, obj);
h = (VoidHand)FldGetTextHandle(fld);
if (h == NULL)
{
h = MemHandleNew (FldGetMaxChars(fld)+1);
ErrFatalDisplayIf(!h, "No Memory");
}
p = (CharPtr)MemHandleLock(h);
StrCopy(p, str);
MemHandleUnlock(h);
FldSetTextHandle(fld, (Handle)h);
}
static Boolean MainFormHandleEvent(EventPtr event)
{
Boolean handled = false;
FieldPtr fld;
switch (event->eType)
{
case frmOpenEvent:
if (event->data.frmOpen.formID == MainForm)
FrmDrawForm(FrmGetFormPtr(MainForm));
handled = true;
break;
case menuEvent:
MenuEraseStatus(NULL);
switch (event->data.menu.itemID)
{
case MainOptionsHelpCmd:
(void)FrmAlert(HelpAlert);
handled = true;
break;
case MainOptionsAboutCmd:
(void)FrmAlert(AboutAlert);
handled = true;
break;
default:
break;
}
break;
case ctlSelectEvent:
switch (event->data.ctlSelect.controlID)
{
case MainCompute:
Go();
handled = true;
break;
default:
break;
}
break;
default:
break;
}
return handled;
}
static Boolean AppHandleEvent(EventPtr event)
{
FormPtr frm;
Int formId;
Boolean handled;
handled = false;
if (event->eType == frmLoadEvent)
{
formId = event->data.frmLoad.formID;
frm = FrmInitForm(formId);
FrmSetActiveForm(frm);
switch (formId)
{
case MainForm:
FrmSetEventHandler(frm, MainFormHandleEvent);
handled = true;
break;
default:
break;
}
}
return handled;
}
static void AppStart()
{
FrmGotoForm(MainForm);
}
static void AppEventLoop(void)
{
EventType event;
short error;
do
{
EvtGetEvent(&event, 100);
if (SysHandleEvent(&event))
continue;
if (MenuHandleEvent((void *)0, &event, &error))
continue;
if (AppHandleEvent(&event))
continue;
FrmDispatchEvent(&event);
}
while (event.eType != appStopEvent);
}
static void AppStop()
{
FrmCloseAllForms();
}
UInt32 PilotMain(UInt16 cmd, MemPtr cmdPBP, UInt16 launchFlags)
{
switch (cmd)
{
case sysAppLaunchCmdNormalLaunch:
AppStart();
AppEventLoop();
AppStop();
break;
default:
break;
}
return 0;
}
Comments
Take a minute to compare this C version of combination
with the versions in other languages. You'll notice that this one,
the Palm SDK version, has a far bigger overhead in terms of lines
of source code. However, once you are used to developing Palm apps,
you'll make your own template out of this overhead, so as to reuse
it for each new application. Who likes to reinvent the wheel?
The core functions are located at the beginning of
the .c file: Factorial(),
Combination() and Go().
The rest of the code encapsulates Palm OS API's, processes events,
manages the event loop and launches the application.
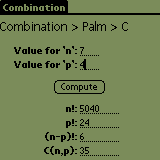
Next sample 
|