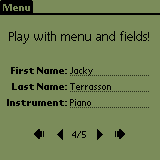
Objective
To design and develop a menu.
Concepts
Hierarchy
Palm OS gives the user the opportunity to tap on a menu button
(or on the menu bar on top of the screen). A menu is made of submenus,
themselves made of items. Each items
gives access to a particular functionality of the application. A
developer is completely free to organize those submenus and items.
However, it is a good idea to follow certain
implicit standards, such as having an Edit
menu containing Cut/Copy/Paste, an Options
menu containing Help/About...
Defining the menu
The menu, its submenus and items will be defined in our .rcp file
with the following structure: MENU contains
PULLDOWNs that contain MENUITEMs
and some SEPARATORs. Here, we have 2
submenus (Edit and Options):
MENU ID MainMenu
BEGIN
PULLDOWN "Edit"
BEGIN
MENUITEM "Cut" ID MainEditCutCmd
"X"
MENUITEM "Copy" ID MainEditCopyCmd
"C"
MENUITEM "Paste" ID MainEditPasteCmd
"V"
MENUITEM SEPARATOR
MENUITEM "Select All" ID MainEditSelectAllCmd
"S"
END
PULLDOWN "Options"
BEGIN
MENUITEM "Help" ID MainOptionsHelpCmd
"H"
MENUITEM SEPARATOR
MENUITEM "About" ID MainOptionsAboutCmd
"A"
END
END
The menu resource we defined in the .rcp file will communicate
with our app thru events and IDs.
If you took the tutorial on forms, you probably remember that this
kind of IDs have to be defined in a .h file that's #included by
both the .rcp and .c components of the app. Menus have to follow
that rule as well:
#define MainMenu 1000
#define MainEditCutCmd 1011
#define MainEditCopyCmd 1012
#define MainEditPasteCmd 1013
#define MainEditSelectAllCmd 1014
#define MainOptionsHelpCmd 1020
#define MainOptionsAboutCmd 1021
Writing code that handles menu events
When the user taps on the menu bar (or on the menu button on the
silkscreen), Palm OS sends a menuEvent
to the application, that has the responsibility to handle it and
take action. The ID of the MENUITEM on which the user tapped is
attached to the event, in event->data.menu.itemID,
so that the app just needs to execute the piece of code corresponding
to the functionality requested by the user.
Here's how we could code a handler for the above menu:
switch (event->data.menu.itemID)
{
case MainEditCutCmd:
...
case MainEditCopyCmd:
...
case
MainEditPasteCmd:
...
case MainEditSelectAllCmd:
...
case MainOptionsHelpCmd:
...
case MainOptionsAboutCmd:
...
default:
...
}
This handler is part of the "endless"
loop started in PilotMain.
Exercise
Download the ZIP file.
In menu.h:
- replace all the question marks with a unique ID
- It is suggested that those IDs be consecutive
and grouped by submenu (starting
at 1000 for pulldown "Record", at 1010 for "Edit",
at 1020 for "Options")
In menu.rcp:
- include the .h
- link the main form with the main menu by adding MENUID
MainMenu into the FORM definition
- add the "Record" pulldown as follows:
PULLDOWN "Record"
BEGIN
MENUITEM "Jarrett" ID MainRecord1Cmd
"1"
MENUITEM "Peacock" ID MainRecord2Cmd
"2"
MENUITEM "De Johnette" ID MainRecord3Cmd
"3"
MENUITEM "Terrasson" ID MainRecord4Cmd
"4"
MENUITEM "Pastorius" ID MainRecord5Cmd
"5"
END
In menu.c:
- include .h file
- in MainFormHandleEvent(), locate the event switch/case menuEvent
block, add MenuEraseStatus(NULL);
- add the handler for the Edit/Clear command:
if (fld = GetFocusObjectPtr())
FldDelete(fld, 0, FldGetTextLength(fld));
- add the handler for Edit/Copy:
if (fld = GetFocusObjectPtr())
FldCopy(fld);
- same for Edit/Cut
if (fld = GetFocusObjectPtr())
FldCut(fld);
- same for Edit/Paste
if (fld = GetFocusObjectPtr())
FldPaste(fld);
- same for Edit/Select All
if (fld = GetFocusObjectPtr())
FldSetSelection(fld, 0, FldGetTextLength(fld));
- same for Options/Help
(void)FrmAlert(HelpAlert);
- same for Options/About
(void)FrmAlert(AboutAlert);
- In AppEventLoop(), add this:
if (MenuHandleEvent((void *)0, &event,
&error))
continue;
Make the app and test it!
Solution
Here's the ZIP file.
And here's how the app should like:
Next topic 
|